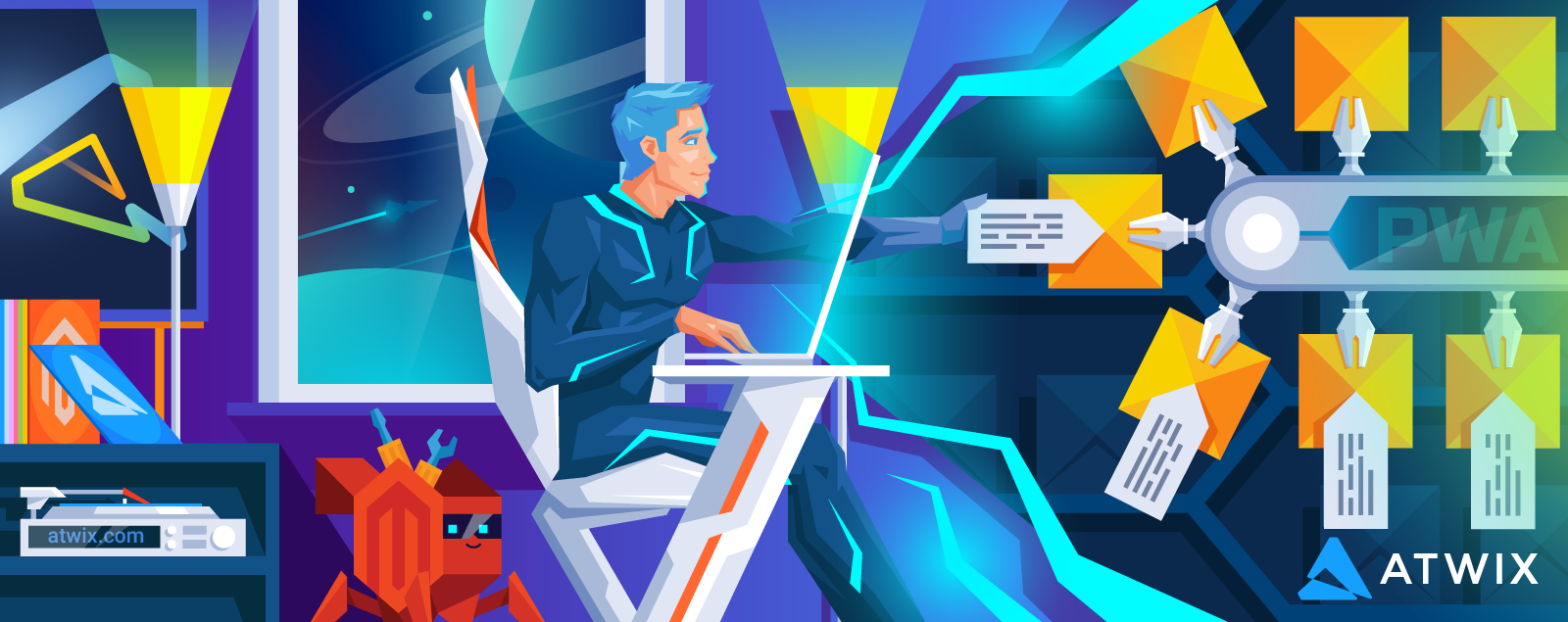
// file: src/components/ProductShortDescription/productShortDescription.js import React, { Fragment } from 'react'; import { useProductShortDescription } from '@theme/talons/ProdutShortDescription/useProductShortDescription'; import { GET_PRODUCT_SHORT_DESCRIPTION } from './productShortDescription.gql'; import { fullPageLoadingIndicator } from '@magento/venia-ui/lib/components/LoadingIndicator'; import RichText from '@magento/venia-ui/lib/components/RichText'; import { string } from 'prop-types'; const ProductShortDescription = props => { const { url_key } = props; const talonProps = useProductShortDescription({ url_key, getProductShortDescription: GET_PRODUCT_SHORT_DESCRIPTION }); const { error, loading, shortDescription } = talonProps; if (error && !shortDescription) { return null; } if (loading) { return fullPageLoadingIndicator } return ( <Fragment> <RichText content={shortDescription} /> </Fragment> ); } ProductShortDescription.propTypes = { url_key: string.isRequired } export default ProductShortDescription;
In order to solve our task, we need to perform two major steps:
// file: webpack.config.js // [...] const path = require('path'); module.exports = async env => { // [...] config.resolve = { ...config.resolve, alias: { ...config.resolve.alias, '@theme': path.resolve(__dirname, 'src') } } // [...] return config; };
Custom Component
We continue the series of articles with technical lifehacks, dedicated to PWA Studio. After the overview of how to Insert CMS Block on the Product Page, we suggest to check out some more interesting situations.
Also, you might notice that the ProductShortDescription
component is imported from some @theme
. That’s our project’s src
directory. It is configured using webpack alias in the project’s webpack.config.js
file. Below you can find the detailed instruction how to make it work.
import ProductShortDescription from '../../../../../../components/ProductShortDescription';
Using the same approach, it is possible to add any other information to the product page, like displaying some custom product attributes, for example. Of course, some adjustments are needed, but that’s not a big deal when almost everything is prepared.
Add Project’s src
dir Alias in Webpack
To assure easier import of the components, we recommend adding the alias. That’s not obligatory, but a good thing to have. With these adjustments in place, we will avoid imports like:
Good luck! And do not hesitate to let your friends and teammates know what you’ve just learned 😉
// file: local-intercept.js function localIntercept(targets) { const {Targetables} = require('@magento/pwa-buildpack'); const targetables = Targetables.using(targets); // load the component to be customized const ProductFullDetailComponent = targetables.reactComponent( '@magento/venia-ui/lib/components/ProductFullDetail/productFullDetail.js' ); // import the custom component in the component to be modified const ProductShortDescription = ProductFullDetailComponent.addImport( "ProductShortDescription from '@theme/components/ProductShortDescription'" ); // insert the custom component that renders product's short description ProductFullDetailComponent.insertAfterJSX( '<section className={classes.title}>', `<${ProductShortDescription} url_key={product.url_key} />` );
} module.exports = localIntercept;
The product’s short description will be inserted to the page after the product’s title. In the ProductFullDetail
component, the title is wrapped by the section <section className={classes.title}>
, which will be taken as a reference to insert the short description.
Imagine the store with PWA Studio storefront, where the Product Page should contain the product’s short description. You may see nothing unusual here, but there is a trick – the default Venia theme doesn’t show the product’s short description by default. Oops. But no worries, that’s not hard to fix. Thanks to PWA Studio’s extensibility framework.
To make things work, we’ll add the adjustments into the project’s local-intercept.js
file. Read what this file is about and how it works in the “Insert CMS Block on the Product Page right before footer” article.